I forget exactly where I originally read about the Godot Engine but I decided to give it a try after I completed my map generator. The verdict: Godot is extremely good. I should note that I have not tried using it for 3D projects, just 2D game projects. Here are some things that stood out to me.
Supported Languages
Godot supports several languages, including C++, C#, D (GDNative), JavaScript and Nim. It even has its own proprietary integrated language, GDScript. A Godot user created a benchmarking tool that helps give an idea of how well each language performs. C# support is still in its early stages, unfortunately, and you can’t use Visual Studio for debugging… Yet! Given some time these functionalities will be added. For now you have to use VS Code to debug, which admittedly isn’t that major of an issue.
Supported Platforms
Windows (and Xbox One by extension), Linux, OSX, iOS, BlackBerry 10 and HTML5 are supported. Other console support cannot be included with Godot as console SDKs are proprietary and all of Godot’s code is open source. There are ways to deal with this limitation, as outlined in the Godot docs.
Organization
Godot and Unity use a lot of similar approaches when it comes to organizing a game project.
Unity uses the entity/component paradigm with scenes and prefabs. Godot instead uses a tree approach to accomplish the same thing. Everything is a tree of nodes. Your scene is a tree containing several nodes for the interface, game elements, and so on. Prefabs are just a tree of nodes. Everything is organized in this manner.
What makes Godot stand out is that there is no distinction between scenes and prefabs like there is with Unity. Scenes and prefabs are the same thing, functionally. If you wanted to, you could instantiate several scenes inside of your main scene. There’s no limitations as to what you are allowed to do.
A GameObject in Unity can have several components, so you have to take a slightly different approach when you want to accomplish the same thing in Godot.
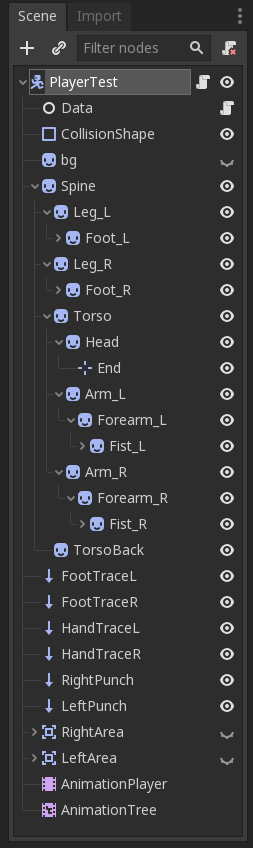
If this were Unity, some of these things would just be components of the base PlayerTest node. Personally i think i prefer Godot’s approach. This way, you can see every component at a glance without having to inspect entities to see which components they contain.
Each node performs a specific task. An AnimatedSprite node renders a sprite and handles its animations, a RigidBody node controls a rigid body, and so-on. When you’re used to being able to have several components on a single GameObject in Unity, it can be a little jarring at first. The upside of Godot’s node system is that it’s very easy to keep track of everything since every component is its own node with whatever name you decide to give it. Instead of having a bunch of components tied to a GameObject, you have a tree of the various nodes your game element requires.
Animation
Godot has an incredible integrated animation system that works for both 2D and 3D projects. The general workflow is:
- Set up your skeletal heirarchy.
- Create animations.
- Use blend trees and state machines to control the current animation state in-game.
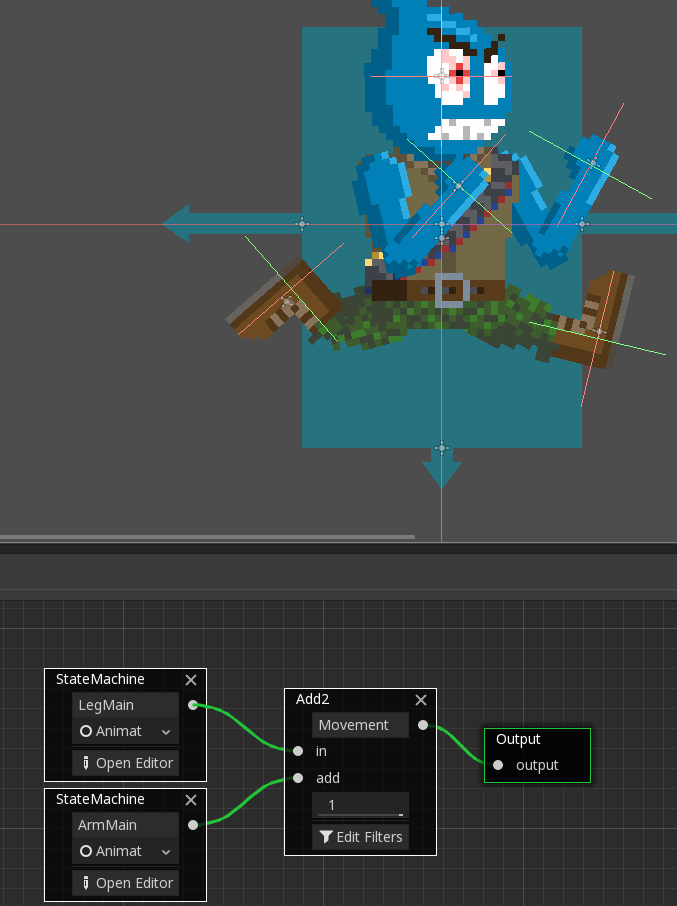
Not only is the animation system intuitive and easy to use, it also allows you to wire function calls to specific keyframes in the animation. It’s been perfectly planned from start to finish.
Workflow
Debugging a project can initially be more tricky with Godot compared to other engines like Unity. With Unity you can set up a scene then run it in debug mode and pause the game so you can browse the active scene in the editor window. This allows you to tweak variables and move things around in the live scene so you can really get an idea for what is causing bugs and how certain elements are interacting.
With Godot you can pause execution and examine the instanced entities in the scene tree but the interactivity isn’t there. You can’t see the active game instances rendered in the editor pane so you can’t dive in and disassemble things like you can with Unity. This is probably my biggest gripe with Godot’s debugging workflow.
Other Considerations
As mentioned before, Godot represents a game scene with a tree of nodes. In Unity you can access a GameObject’s component pretty easily. How do you do this in Godot when your components are organized in a tree structure?
var list = GetNode<VBoxContainer>("CenterContainer/Units/UnitContainer");
Nodes in Godot are organized visually as a tree, but when accessing them via code you use a string path.
I ended up writing some utility functions for creating nodes via code since it tends to get a little obnoxious without them.
public static T Instantiate<T>(string path) where T : Node { string temp = "res://Prefabs/#.tscn"; string filepath = temp.Replace("#", path); var node = GD.Load<PackedScene>(filepath); T inst = (T)node.Instance(); return inst; } ... // Usage example var player = Util.Instantiate<PlayerController>("Characters/PlayerTest");
Conclusion
Godot is shaping up to be the first open source game engine that is on par with existing game engines. Even in its current state i’d consider it a viable alternative to other existing 2D game engines. As it matures i expect it will get more and more attention.